API access
Outreach currently enables access to the REST API via API calls authenticated via OAuth 2.0 protocol. Additionally a limited set of API endpoints is available with an authentication token you can obtain through a proprietary S2S protocol.OAuth in a nutshell
OAuth 2.0 is a standard authorization protocol and there is a lot of information online on how to implement it. Here is how it works in a nutshell:
- Once you need to connect to Outreach in your own application, you redirect the user to an URL like https://api.outreach.io/oauth/authorize?client_id=...&redirect_uri=...&response_type=code&scope=....
- At the authorization page user is informed about which data your application intends to use and gives their consent to do so.
- Following the consent Outreach redirects the user to the URL on your server passing along a
code
query parameter also known as the Authorization Grant. - You use the Authorization Grant to obtain the Access Token.
- With the Access Token you call the Outreach REST API endpoints. Outreach will execute all the calls on behalf of the user who performed authorization.
- Access token is valid for limited period only but you can refresh it using the refresh token returned in the same response.
Setting up OAuth
To begin using the REST API you need to create an Outreach app. Then go to the API access tab to configure access specifics. You'll need to specify one or more redirect URI's and select the OAuth data scopes that your application intends to use. Please select at least one scope and specify at least one redirect URI.
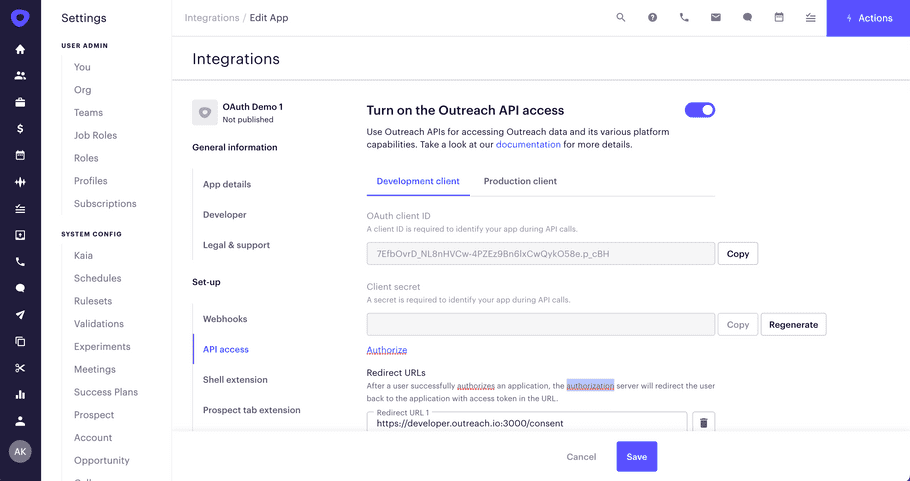
OAuth credentials
For each Outreach app, the development and production credentials are generated.
- Development credentials are provisioned immediately and are intended to be used during application development and testing. Any change (e.g. to scopes) is applied immediately on save. The authorization page warns users that they are using development and unreviewed application and usage of development version is limited. Do not let your end-users use these development credentials.
- Production credentials are provisioned after the app goes through the publishing process and eventually a review. The scopes and URLs are updated when you click on the Publish button.
Please be aware that OAuth client secrets will be displayed only once, when generated. You cannot display them again. Use the "Regenerate" button to create new secrets if necessary.
Limitations of development credentials
If an end-user goes through OAuth flow that contains development credentials they will be warned that the application is not production ready.
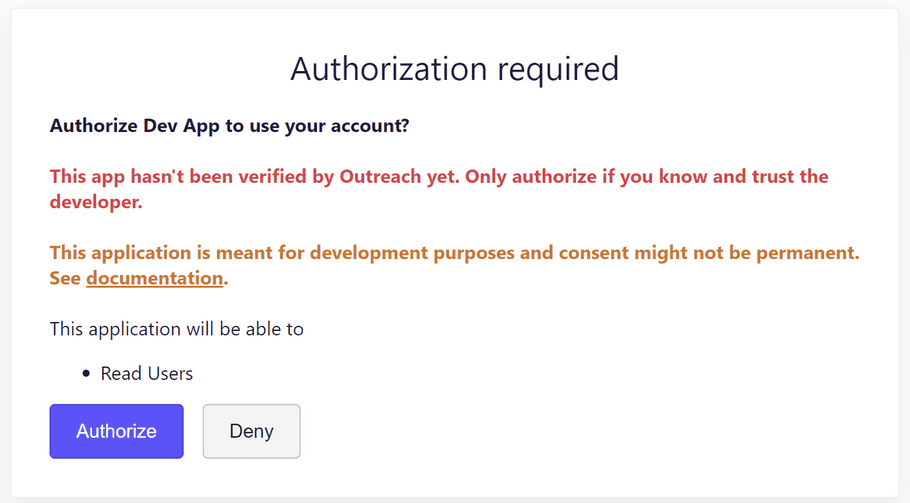
Application's development credentials can be authorized by any user from any org. However, the development credentials are meant for developers and testers only. We do not impose any arbitrary block to enforce fair usage but we expire the grant provided by users and revoke all tokens, so users need to re-authorize the app once in a while.
Development credentials are usable with no limitation for up to 10 people from the organization that owns the app.
Users from other orgs or when there are more users from the owning org will need to reauthorize the app weekly.
Requesting access token
Following user authorization browser will redirect the user to the specified redirect URI containing the authorization grant:<redirect_uri>?code=<authorization_grant>
.
Read the value of the code
parameter and pass it to https://api.outreach.io/oauth/token
to get your OAuth token for this user:curl https://api.outreach.io/oauth/token \
-X POST \
-d client_id=<client_id> \
-d client_secret=<client_secret> \
-d redirect_uri=<redirect_uri> \
-d grant_type=authorization_code \
-d code=<authorization_grant>
Please be aware that the secret might contain URL-unsafe characters and must be properly encoded. Some tools are known to properly encode redirect URL but not the secret.
This call will only be successful if all of the parameter values match exactly the values which initiated this OAuth flow.
In some situations you may need to set up different redirect URIs for development and production client IDs so that you know which credential (prod or dev) you need to use in the above call.
Alternatively you can use thestate
query parameter when initiating the OAuth flow. For example the flow initiated with: https://api.outreach.io/oauth/authorize?client_id=<client_id>&redirect_uri=<redirect_uri>&response_type=code&scope=<scope1+scope2>&state=dev
<redirect_uri>?code=<authorization_grant>&state=dev
. You can then read the value of the state parameter and choose the correct credential for the token acquisition call.The response of /oauth/token
looks like{
"access_token": "eyJhbGc...",
"token_type": "Bearer",
"expires_in": 7200,
"refresh_token": "EcC-5gWjbN...",
"scope": "users.read",
"created_at": 1706289101
}
Refreshing access token
You should store the refresh token securely and use it to obtain a new access token once the original expires. Call/oauth/token
as above but use grant_type=refresh_token
.curl https://api.outreach.io/oauth/token \
-X POST \
-d client_id=<client_id> \
-d client_secret=<client_secret> \
-d grant_type=refresh_token \
-d refresh_token=<refresh_token>