Custom Objects via API
Custom Objects are a way to store custom data in Outreach. You can use Custom Objects to store data that is not natively supported by Outreach, such as product information, customer data, or any other custom data that you need to store. They are configurable in the Outreach Application. The public API allows you to list, get, create, update and delete custom object records.
Accessing Custom Object Endpoints
In Outreach API, custom objects are under their specific pathsapi/v2/customObjects
. The custom objects and their fields are identifier by their internal name that
your administrator had chosen when configuring the object in the Outreach Application. That also means that when a relationship refers
to a custom object its type is the internal name of the referred custom object.
As for standard resources, they have their own API Reference documentation. It is accessible through an authenticated portal. This documentation is automatically generated and uploaded daily based on the custom object schemas built by your administrators. Therefore you may expect a delay between a new custom object creation or a change to its configuration and its availability in the API Reference (maximum 1 day).Without delay, you can find all the custom objects with their attributes and relationships by calling the schema endpoint. They will be present next to standard resources.
GET https://api.outreach.io/api/v2/schema
{
"$schema": "http://json-schema.org/draft-07/hyper-schema#",
"definitions": {
"account": {
"$schema": "http://json-schema.org/draft-07/hyper-schema#",
"definitions": {
"name": {
"filterable": true,
"sortable": true,
"type": "string"
},
// ...
},
// ...
"stability": "prototype",
"type": "object"
},
"myCustomObject": {
"$schema": "http://json-schema.org/draft-07/hyper-schema#",
"definitions": {
"my_object_name": {
"filterable": true,
"sortable": true,
"type": "string"
},
// ...
},
// ...
"stability": "prototype",
"type": "object"
}
}
}
Note: the schema endpoint works for unauthenticated users too. If the request is not authenticated, the schema will not contain your organization's custom objects.
CRUD operations on custom objects
Listing, getting, creating, updating and deleting custom object records is done in a similar way to standard resources. Let's assume a custom object with internal namevehicles
was defined using Outreach Application.
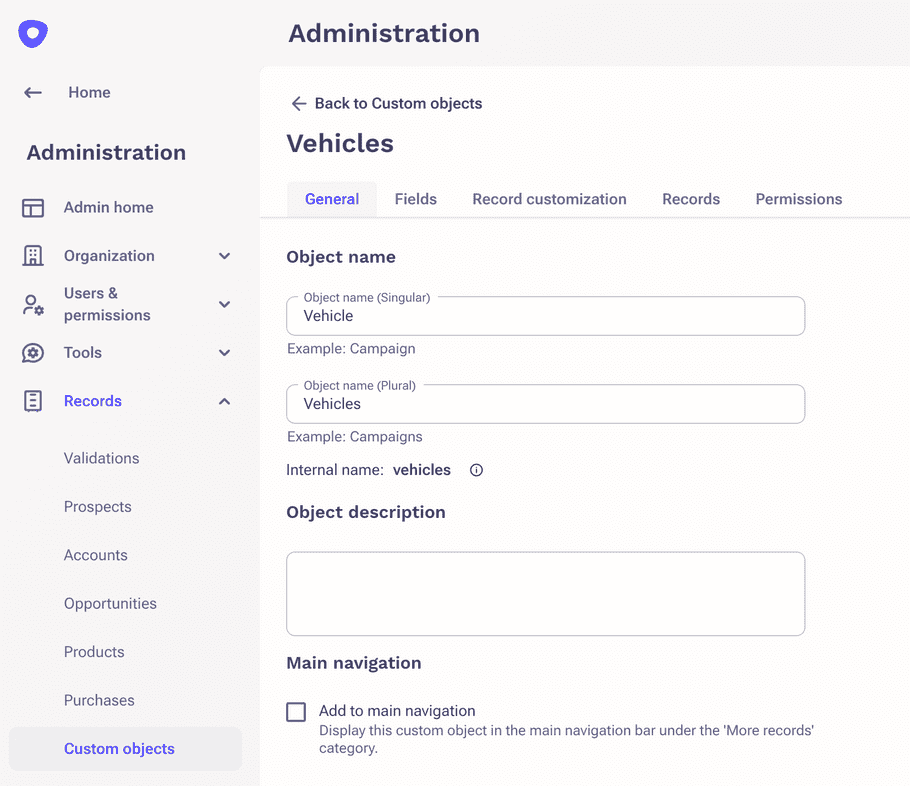
main_driver
.
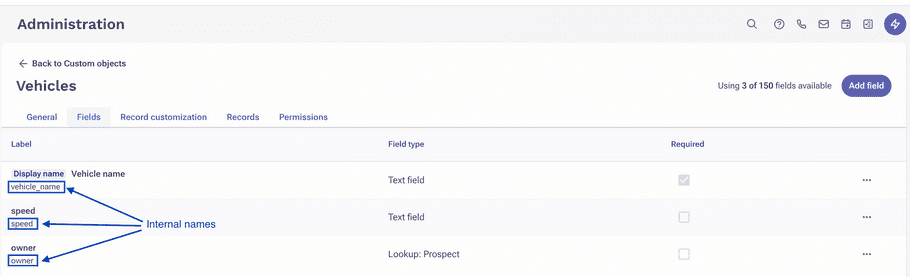
Here are the endpoints available:
- List All Custom Object Records, response includes pagination as standard resources.
GET https://api.outreach.io/api/v2/customObjects/vehicles
{
"data": [
{
"type": "vehicles",
"id": 123456,
"attributes": {
"createdAt": "2024-07-25T13:18:30.736332Z",
"vehicle_name": "DeLorean",
"speed": "130mph",
"updatedAt": "2024-07-25T13:18:30.736332Z"
},
"relationships": {
"main_driver": {
"data": {
"type": "prospect",
"id": 123
}
}
},
"links": {
"self": "https://api.outreach-staging.com/api/v2/customObjects/vehicles/123456"
}
},
// ...
],
"meta": {
"count": 10,
"count_truncated": false
},
"links": {
"first": "https://api.outreach-staging.com/api/v2/customObjects/vehicles?page%5Bsize%5D=50",
"next": "https://api.outreach-staging.com/api/v2/customObjects/vehicles?page%5Bafter%5D=Wzk3Mjc1XQ%3D%3D&page%5Bsize%5D=50"
}
}
- Get a Custom Object Record
GET https://api.outreach.io/api/v2/customObjects/vehicles/123456
{
"data": {
"type": "special_customers",
"id": 123456,
"attributes": {
// ...
},
"relationships": {
// ...
},
"links": {
"self": "https://api.outreach-staging.com/api/v2/customObjects/vehicles/123456"
}
},
}
- Create a Custom Object Record (similar response as for GET one)
POST https://api.outreach.io/api/v2/customObjects/vehicles
with request body
{
"data": {
"type": "vehicles",
"attributes": {
"vehicle_name": "Ford T",
"speed": "45mph"
},
"relationships": {
"main_driver": {
"data": {
"type": "prospect",
"id": 4321
}
}
}
}
}
- Update a Custom Object Record (similar response as for GET one)
PATCH https://api.outreach.io/api/v2/customObjects/vehicles/123456
with request body
{
"data": {
"type": "vehicles",
"id": 123456,
"attributes": {
"speed": "117mph"
}
}
}
- Delete a Custom Object Record (response is empty, HTTP status 204)
DELETE https://api.outreach.io/api/v2/customObjects/vehicles/123456
Filtering and sorting
On the LIST endpoints you can use filters and sort in a similar way as for standard resources. The filters are based on the fields of the custom object.
GET https://api.outreach.io/api/v2/customObjects/vehicles?filter[age]=0..10&filter[main_driver][id]=123&sort=-vehicle_name
One important point is when filtering by relationship, as for standard object, you can always filter by the referred object id. You can also target different attributes depending and the type of the relationship:
- for prospect, opportunity, account: name.
- for user: email and name.
- for custom objects: the field marked as display name.
vehicles
custom object has a reference to a custom object vehicle_brands
with a field name
marked as display name.
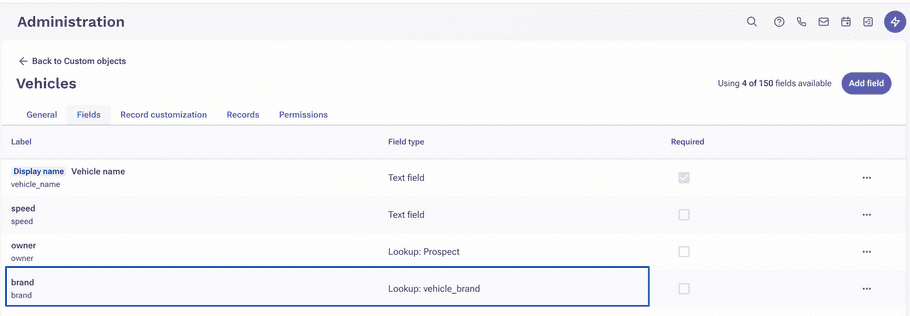
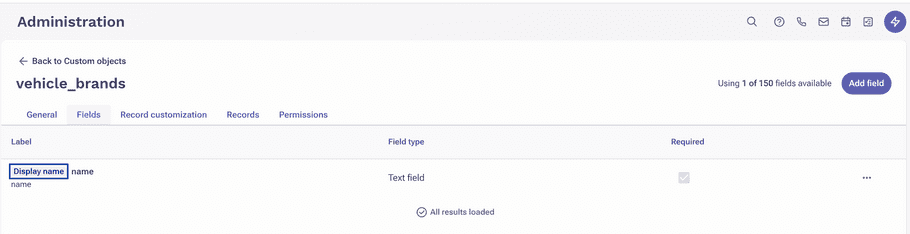
LIST https://api.outreach.io/api/v2/customObjects/vehicles?filter[brand][name]=DMC
{
"data": [
{
"type": "vehicles",
"id": 123456,
"attributes": {
"createdAt": "2024-07-25T13:18:30.736332Z",
"vehicle_name": "DeLorean",
"speed": "117mph",
"updatedAt": "2024-07-25T13:18:30.736332Z"
},
"relationships": {
"main_driver": {
"data": {
"type": "prospect",
"id": 123
}
},
"brand": {
"data": {
"type": "vehicle_brands",
"id": 1
}
}
},
"links": {
"self": "https://api.outreach-staging.com/api/v2/customObjects/vehicles/123456"
}
}
],
"meta": {
"count": 1,
"count_truncated": false
},
"links": {
"first": "https://api.outreach-staging.com/api/v2/customObjects/vehicles?page%5Bsize%5D=50?filter[brand][name]=DMC",
}
}
vehicle_brands
changes and has a different display name, the filter will not work anymore. You need to use the field newly marked as display name. If you would like the filter to work regardless of the display name, you need to filter by the id of vehicle_brands
.Including Related Resources
Make sure to read about including related resources in APIv2. Similarly to standard object, to include a resource you will be using the reference field internal name. In the case ofvehicles
where main_driver
is a reference to a prospect, you would do:GET https://api.outreach.io/api/v2/customObjects/vehicles/123456?include=main_driver
{
"data": {
"type": "vehicles",
"id": 123456,
"attributes": {
"createdAt": "2024-07-25T13:18:30.736332Z",
"vehicle_name": "DeLorean",
"speed": "117mph",
"updatedAt": "2024-07-25T13:18:30.736332Z"
},
"relationships": {
"main_driver": {
"data": {
"type": "prospect",
"id": 123
}
},
"brand": {
"data": {
"type": "vehicle_brands",
"id": 1
}
}
},
"links": {
"self": "https://api.outreach-staging.com/api/v2/customObjects/vehicles/123456"
}
},
"included": [
{
"type": "prospect",
"id": 123,
"attributes": {
"name": "John"
}
}
]
}
You can only go one level deep to include related resources that are custom objects. That means that the following would not work:
GET https://api.outreach.io/api/v2/customObjects/vehicles?include=brand.another_object
Meanwhile this would:
GET https://api.outreach.io/api/v2/customObjects/vehicles?include=main_driver.account
Sparse Fieldsets
Make sure to read about sparse fieldsets in APIv2 . Custom objects support sparse fieldsets in the same way as standard resources.Here you target resources by their type (internal name of the custom object) and the fields by their internal name.
GET https://api.outreach.io/api/v2/customObjects/vehicles/123456?include=brand&fields[vehicle_brands]=name&fields[vehicles]=name
{
"data":
{
"type": "campaigns",
"id": 123456,
"attributes": {
"name": "DeLorean"
}
},
"included": [
{
"type": "vehicle_brands",
"id": 1,
"attributes": {
"name": "DMC"
}
}
]
}